what does Empty File after upload covert.io
How to process file uploads in Go
Processing user uploaded files is a common task in web development and it'due south quite likely that y'all'll need to develop a service that handles this job from time to time. This article volition guide you through the process of handling file uploads on a Get spider web server and talk over common requirements such equally multiple file uploads, progress reporting, and restricting file sizes.
In this tutorial, we will take a look at file uploads in Go and cover common requirements such as setting size limits, file type restrictions, and progress reporting. You can find the full source code for this tutorial on GitHub.
Getting started
Clone this repository to your computer and cd
into the created directory. You'll see a main.get
file which contains the following code:
package main import ( "log" "internet/http" ) func indexHandler ( w http . ResponseWriter , r * http . Asking ) { w . Header (). Add ( "Content-Type" , "text/html" ) http . ServeFile ( w , r , "index.html" ) } func uploadHandler ( w http . ResponseWriter , r * http . Request ) { if r . Method != "POST" { http . Fault ( w , "Method not allowed" , http . StatusMethodNotAllowed ) return } } func primary () { mux := http . NewServeMux () mux . HandleFunc ( "/" , indexHandler ) mux . HandleFunc ( "/upload" , uploadHandler ) if err := http . ListenAndServe ( ":4500" , mux ); err != nothing { log . Fatal ( err ) } }
The code hither is used to get-go a server on port 4500 and return the index.html
file on the root route. In the index.html
file, nosotros have a form containing a file input which posts to an /upload
route on the server.
<!DOCTYPE html> < html lang = "en" > < head > < meta charset = "UTF-eight" /> < meta name = "viewport" content = "width=device-width, initial-scale=1.0" /> < meta http-equiv = "X-UA-Compatible" content = "ie=edge" /> < championship >File upload demo</ title > </ caput > < body > < course id = "form" enctype = "multipart/form-data" action = "/upload" method = "Post" > < input class = "input file-input" type = "file" proper noun = "file" multiple /> < push class = "button" type = "submit" >Submit</ push > </ class > </ body > </ html >
Let's go ahead and write the lawmaking nosotros need to procedure file uploads from the browser.
Set the maximum file size
It'southward necessary to restrict the maximum size of file uploads to avert a situation where clients accidentally or maliciously upload gigantic files and end up wasting server resources. In this department, nosotros'll set a maximum upload limit of One Megabyte and show an error if the uploaded file is greater than the limit.
A mutual approach is to check the Content-Length
request header and compare to the maximum file size allowed to meet if it'southward exceeded or not.
if r . ContentLength > MAX_UPLOAD_SIZE { http . Error ( due west , "The uploaded image is too big. Please use an image less than 1MB in size" , http . StatusBadRequest ) return }
I don't recommended using this method considering the Content-Length
header can be modified on the client to be whatever value regardless of the actual file size. It'due south ameliorate to rely on the http.MaxBytesReader method demonstrated beneath. Update your main.go
file with the highlighted portion of the following snippet:
const MAX_UPLOAD_SIZE = 1024 * 1024 // 1MB func uploadHandler ( w http . ResponseWriter , r * http . Request ) { if r . Method != "Post" { http . Fault ( w , "Method not allowed" , http . StatusMethodNotAllowed ) return } r . Body = http . MaxBytesReader ( west , r . Body , MAX_UPLOAD_SIZE ) if err := r . ParseMultipartForm ( MAX_UPLOAD_SIZE ); err != zilch { http . Error ( westward , "The uploaded file is too large. Delight choose an file that'southward less than 1MB in size" , http . StatusBadRequest ) render } }
The http.MaxBytesReader()
method is used to limit the size of incoming asking bodies. For single file uploads, limiting the size of the request torso provides a adept approximation of limiting the file size. The ParseMultipartForm()
method afterwards parses the asking body every bit multipart/form-data
up to the max memory argument. If the uploaded file is larger than the argument to ParseMultipartForm()
, an error volition occur.
Relieve the uploaded file
Next, let's call back and save the uploaded file to the filesystem. Add the highlighted portion of code snippet below to the cease of the uploadHandler()
function:
func uploadHandler ( west http . ResponseWriter , r * http . Asking ) { // truncated for brevity // The statement to FormFile must match the name attribute // of the file input on the frontend file , fileHeader , err := r . FormFile ( "file" ) if err != nil { http . Error ( w , err . Fault (), http . StatusBadRequest ) return } defer file . Close () // Create the uploads folder if it doesn't // already exist err = bone . MkdirAll ( "./uploads" , bone . ModePerm ) if err != zilch { http . Fault ( w , err . Mistake (), http . StatusInternalServerError ) return } // Create a new file in the uploads directory dst , err := os . Create ( fmt . Sprintf ( "./uploads/%d%due south" , fourth dimension . Now (). UnixNano (), filepath . Ext ( fileHeader . Filename ))) if err != nil { http . Error ( w , err . Error (), http . StatusInternalServerError ) return } defer dst . Close () // Copy the uploaded file to the filesystem // at the specified destination _ , err = io . Copy ( dst , file ) if err != zippo { http . Mistake ( due west , err . Error (), http . StatusInternalServerError ) return } fmt . Fprintf ( w , "Upload successful" ) }
Restrict the blazon of the uploaded file
Let's say we want to limit the type of uploaded files to just images, and specifically but JPEG and PNG images. We demand to detect the MIME blazon of the uploaded file then compare information technology to the allowed MIME types to make up one's mind if the server should continue processing the upload.
You can use the accept
aspect in the file input to define the file types that should be accepted, merely you yet need to double check on the server to ensure that the input has not been tampered with. Add together the highlighted portion of the snippet below to the FileHandlerUpload
office:
func uploadHandler ( west http . ResponseWriter , r * http . Request ) { // truncated for brevity file , fileHeader , err := r . FormFile ( "file" ) if err != nil { http . Error ( w , err . Error (), http . StatusBadRequest ) return } defer file . Close () buff := make ([] byte , 512 ) _ , err = file . Read ( buff ) if err != nil { http . Error ( due west , err . Error (), http . StatusInternalServerError ) render } filetype := http . DetectContentType ( buff ) if filetype != "image/jpeg" && filetype != "image/png" { { http . Fault ( due west , "The provided file format is not allowed. Please upload a JPEG or PNG paradigm" , http . StatusBadRequest ) return } _ , err := file . Seek ( 0 , io . SeekStart ) if err != zip { http . Mistake ( w , err . Error (), http . StatusInternalServerError ) return } // truncated for brevity }
The DetectContentType()
method is provided by the http
packet for the purpose of detecting the content blazon of the given information. It considers (at most) the commencement 512 bytes of data to decide the MIME type. This is why nosotros read the offset 512 bytes of the file to an empty buffer before passing it to the DetectContentType()
method. If the resulting filetype
is neither a JPEG or PNG, an fault is returned.
When we read the beginning 512 bytes of the uploaded file in club to decide the content type, the underlying file stream pointer moves forward by 512 bytes. When io.Copy()
is chosen later on, information technology continues reading from that position resulting in a corrupted image file. The file.Seek()
method is used to return the pointer back to the commencement of the file so that io.Copy()
starts from the beginning.
Handle multiple files
If you lot want to handle the case where multiple files are beingness sent from the client at once, you can manually parse and iterate over each file instead of using FormFile()
. After opening the file, the rest of the code is the same as for unmarried file uploads.
func uploadHandler ( w http . ResponseWriter , r * http . Request ) { if r . Method != "POST" { http . Mistake ( w , "Method not allowed" , http . StatusMethodNotAllowed ) return } // 32 MB is the default used past FormFile() if err := r . ParseMultipartForm ( 32 << 20 ); err != nil { http . Error ( w , err . Mistake (), http . StatusInternalServerError ) render } // Go a reference to the fileHeaders. // They are accessible simply after ParseMultipartForm is called files := r . MultipartForm . File [ "file" ] for _ , fileHeader := range files { // Restrict the size of each uploaded file to 1MB. // To foreclose the amass size from exceeding // a specified value, use the http.MaxBytesReader() method // before calling ParseMultipartForm() if fileHeader . Size > MAX_UPLOAD_SIZE { http . Mistake ( westward , fmt . Sprintf ( "The uploaded image is likewise big: %due south. Please employ an prototype less than 1MB in size" , fileHeader . Filename ), http . StatusBadRequest ) render } // Open up the file file , err := fileHeader . Open () if err != goose egg { http . Error ( due west , err . Error (), http . StatusInternalServerError ) render } defer file . Close () vitrify := make ([] byte , 512 ) _ , err = file . Read ( buff ) if err != nothing { http . Error ( w , err . Error (), http . StatusInternalServerError ) return } filetype := http . DetectContentType ( buff ) if filetype != "image/jpeg" && filetype != "image/png" { http . Error ( w , "The provided file format is not immune. Please upload a JPEG or PNG image" , http . StatusBadRequest ) return } _ , err = file . Seek ( 0 , io . SeekStart ) if err != nil { http . Error ( due west , err . Error (), http . StatusInternalServerError ) return } err = os . MkdirAll ( "./uploads" , bone . ModePerm ) if err != nil { http . Mistake ( w , err . Error (), http . StatusInternalServerError ) render } f , err := os . Create ( fmt . Sprintf ( "./uploads/%d%s" , time . Now (). UnixNano (), filepath . Ext ( fileHeader . Filename ))) if err != aught { http . Error ( westward , err . Fault (), http . StatusBadRequest ) return } defer f . Close () _ , err = io . Copy ( f , file ) if err != zippo { http . Mistake ( west , err . Fault (), http . StatusBadRequest ) return } } fmt . Fprintf ( w , "Upload successful" ) }
Study the upload progress
Next, let's add progress reporting of the file upload. We can make use of the io.TeeReader()
method to count the number bytes read from an io.Reader
(in this case each file). Here'due south how:
// Progress is used to track the progress of a file upload. // Information technology implements the io.Writer interface so it tin exist passed // to an io.TeeReader() type Progress struct { TotalSize int64 BytesRead int64 } // Write is used to satisfy the io.Author interface. // Instead of writing somewhere, it simply aggregates // the total bytes on each read func ( pr * Progress ) Write ( p [] byte ) ( due north int , err error ) { n , err = len ( p ), nothing pr . BytesRead += int64 ( n ) pr . Impress () return } // Print displays the current progress of the file upload // each time Write is called func ( pr * Progress ) Print () { if pr . BytesRead == pr . TotalSize { fmt . Println ( "Done!" ) return } fmt . Printf ( "File upload in progress: %d\due north" , pr . BytesRead ) } func uploadHandler ( due west http . ResponseWriter , r * http . Request ) { // truncated for brevity for _ , fileHeader := range files { // [..] pr := & Progress { TotalSize : fileHeader . Size , } _ , err = io . Copy ( f , io . TeeReader ( file , pr )) if err != nil { http . Error ( w , err . Error (), http . StatusBadRequest ) render } } fmt . Fprintf ( westward , "Upload successful" ) }
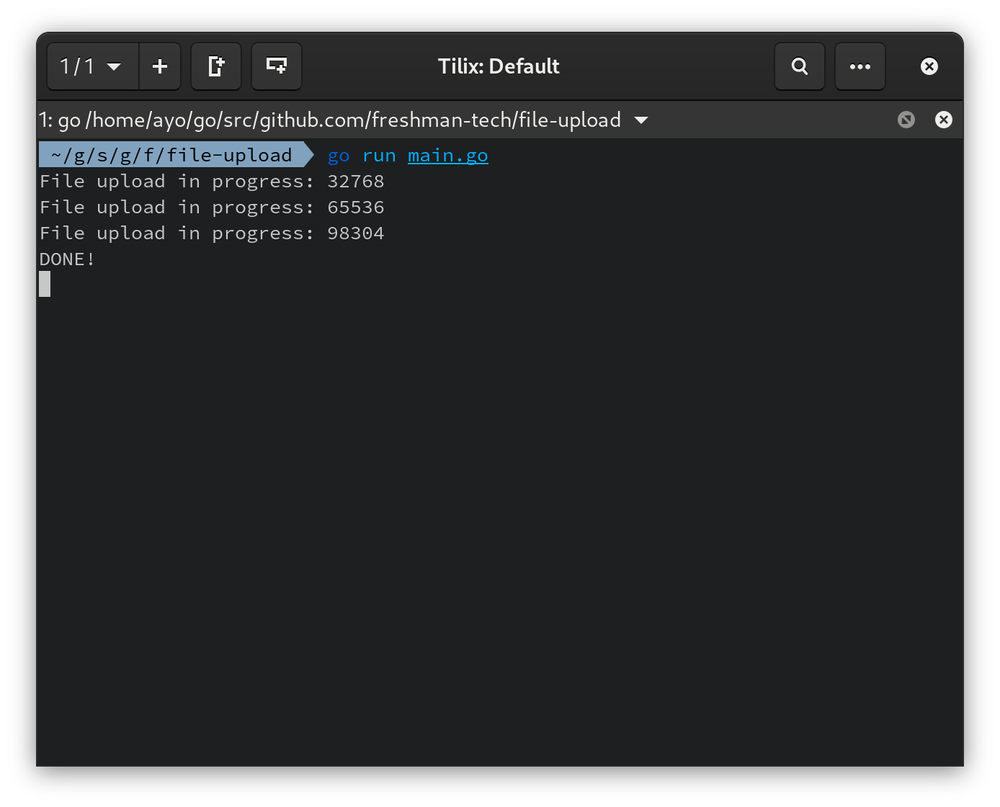
Conclusion
This wraps upwards our effort to handle file uploads in Go. Don't forget to grab the full source lawmaking for this tutorial on GitHub. If you lot have whatever questions or suggestions, feel free to leave a comment below.
Thanks for reading, and happy coding!
Source: https://freshman.tech/file-upload-golang/
0 Response to "what does Empty File after upload covert.io"
Post a Comment